PHPRad Framework at a Glance
Getting Started
This document is intended to introduce you to the PHPRad framework and the principles of MVC architecture.
The document will show you step-by-step of creating both static and dynamic page application using some basic principles of MVC Framework.
Separation of User Actions, Busines Login, And Views
One of the benefits of using a MVC methodology is that it helps enforce a clean separation of concerns between the models, views and controllers within an application. Maintaining a clean separation of concerns makes the developing, maintaining and testing of large complex applications much easier, since the contract between different application components are more clearly defined and articulated.
It helps in building reusable components
it employs DRY mechanism
This Makes it Easier for different Personel such as (UI Designer, Database Developer, System Engineer) to work on the same project without any conflict of interest.
Why PHPRad
Simple Flexible Framework
PHPRad is a development enviroment for building web applications easier and fasterLight Weight
The core system requires only a few very small libraries. This is in stark contrast to many frameworks that requirePHPRad is Smartly Packed
PHPRad comes with libraries and function helpers that enables you accomplish come basic task.PHPRad Uses MVC
PHPRad uses the Model-View-Controller approach, which allows great separationGenerate SEO & Human Readable URLs
The URLs generated by PHPRad are Human Readable and search-engine friendlyPHPRad is Free
PHPRad is licensed under the MIT license so you can do what ever you want to do with it.No Template Engines
You don't have to learn new template engine just to waste time in building application that is laggyWell Documented
Every Function and Class in PHPRad are well structured and professionally Commented Code.
Easy to Extend
PHPRad Framework can easily be extended with other external libraries and packagesSome Basic Features and Functionalities PHPRad
Here are some of the main basic functionalities and feature which PHPRad comes with.- MVC Framework
- Light Weight
- Agnostics database Support.
- MYsqli ORM Feature
- Form and Data Validation
- Security Features (CSRF,XSS,SQL Injection) ETC...
- Session Management
- Email Sending Class. (SMTP, and Mail)
- Image Manipulation Library (cropping, resizing, etc.)
- File Uploading Class
- Pagination
- Resource Caching
- Error Logging
- Mobile Platform Support
- Search-engine Friendly URLs
- Flexible URI Routing
- Class Extensions
- Helper functions
System Requirments
PHP 5.4+
PHPRad supports PHP version 5.4 or later. Builds are running continuously on 5.4,5.6, 7.0 and 7.1
HTTP Server
Apache is supported with mod_rewrite enabled. PHPRad can run on different web server.
Database (optional)
PHPRad is database agnostic, that Means you can use any type of database you want. By Default Mysqli ORM is included.
Downloading And Installing PHPRad MVC Framework
- First, download the framework, either directly or by cloning the repo from GitHub.
- Open App/Config.php and enter your database configuration data.
- Add controllers, views and models.
Lol. That's All
Framework Application Structure
Project Directory
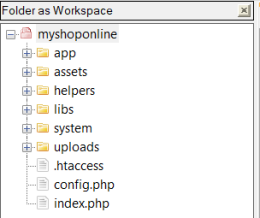
Expanded Project Directory
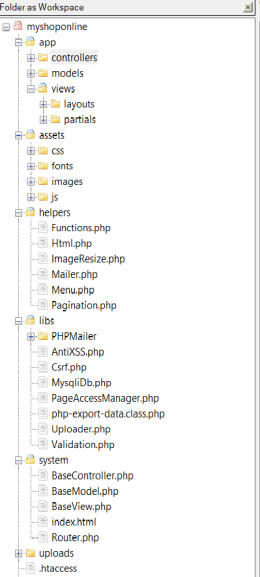
Project Directories Descriptions
-
app Application Specifics Files and Classes
controllers Contains Page Controller Classes
models Contain Page Models Classes
views
layouts Contains Shared Layout Views
partials Contain Page Views
-
assests Public Files and Contents
css Contains css files
fonts Contain fonts and icon fonts
images contain Images and icon images
js Contain javascript files and plugins
-
helpers Contain class and libraries helpers functions
-
libs Contain Libraries
-
system Contain framework core classes
-
htaccess mod_rewrite file
-
config.php Application Configuration Settings
-
index.php Application Entry Point
PHPRad Application Configuration Constants (config.php)
define('DEVELOPMENT_MODE',true);// set to false if in p
define('ROOT', str_replace('\\', '/', dirname(__FILE__)) . '/');
define('ROOT_DIR_NAME',basename(ROOT));
define('SITE_NAME',"emman");
define('APP_ID',"0bced9ce0c7fd29c6d97996b685dfed1");
define('SITE_ADDR',$site_url);
define('META_THEME_COLOR',"#000000"); //Application Default Color (Mostly Used By Mobile)
// Application Files and Directories
define('IMG_DIR', "assets/images/");
define('SITE_FAVICON',IMG_DIR . "favicon.png");
define('SITE_LOGO',IMG_DIR . "logo.png");
define('CSS_DIR',SITE_ADDR . "assets/css/");
define('JS_DIR',SITE_ADDR . "assets/js/");
define('APP_DIR',"app/");
define('SYSTEM_DIR',ROOT . 'system/');
define('HELPERS_DIR',ROOT . 'helpers/');
define('LIBS_DIR',ROOT . 'libs/');
define('MODELS_DIR',APP_DIR . "models/");
define('CONTROLLERS_DIR',APP_DIR . 'controllers/');
define('VIEWS_DIR',APP_DIR . 'views/');
define('LAYOUTS_DIR',VIEWS_DIR . 'layouts/');
define('PAGES_DIR',VIEWS_DIR . 'partials/');
// File Upload Directories
define('UPLOAD_DIR', 'uploads/');
define('UPLOAD_FILE_DIR',UPLOAD_DIR . 'files/');
define('UPLOAD_IMG_DIR', UPLOAD_DIR . 'photos/');
define('MAX_UPLOAD_FILESIZE',trim(ini_get('upload_max_filesize')));
// Application Page Settings
define("DEFAULT_PAGE","index"); //Default Controller Class
define("DEFAULT_PAGE_ACTION","index"); //Default Controller Action
define('DEFAULT_LAYOUT',LAYOUTS_DIR . 'default_layout.php');
define('HOME_PAGE','home');
// Page Meta Information
define('META_AUTHOR','');
define('META_DESCRIPTION','');
define('META_KEYWORDS','');
define('META_VIEWPORT','width=device-width, initial-scale=1.0');
// Email Configuration Default Settings
define('USE_SMTP',false);
define('SMTP_USERNAME','');
define('SMTP_PASSWORD','');
define('SMTP_HOST','');
define('SMTP_PORT','');
//Default Email Sender Details. Please set this even if you are not using SMTP
define('DEFAULT_EMAIL','');
define('DEFAULT_EMAIL_ACCOUNT_NAME','');
// Database Configuration Settings
define('DB_HOST','localhost');
define('DB_USERNAME','root');
define('DB_PASSWORD','');
define('DB_NAME','myshoponline_db');
define('MAX_RECORD_COUNT',20); //Default Max Records to Retrieve per Page
define('ORDER_TYPE','DESC'); //Default Order Type
Application Entry Point (Index.php)
Mod_Rewrite (.htaccess)
By default Apache server has mod_rewrite enabled, if not mod_rewrite needs to be enabled.
The .htaccess contains the rules for the mod_rewrite which direct all request to the index page except if the request is an actual file or a directory.()
Example:RewriteEngine on
RewriteCond %{REQUEST_FILENAME} !-f
RewriteCond %{REQUEST_FILENAME} !-d
RewriteRule ^(.*)$ index.php?request_uri=$1 [NC,L,QSA,B]
Autoloading
You don't want to manually include or require class file that we need in every script in the project, that's why PHP MVC frameworks have this autoloading feature. For example, if you put your own class file under 'lib' folder, then it will be auto loaded. that means you can instantiate the class and use it witout requiring the class file.// Register Autoloaders
spl_autoload_register("autoloadModel");
spl_autoload_register("autoloadController");
spl_autoload_register("autoloadLibrary");
spl_autoload_register("autoloadHelper");
Router Dispatch
$page=new Router;
$page->init(); // Bootstrap Page
Routing
The Router translates URLs into controllers and actions and actions arguments.PHPRad URLs
By default, URLs in PHPRad are designed to be search-engine and
human readable. Rather than using the traditional query string
approach
to URLs that is used to build dynamic systems, PHPRad uses a
path-based mechanism:
http://localhost/myshop/controller/action/arg1/arg2/arg3/arg4/...
URI Segments
The segments in the URL, in following with the PHPRad MVC approach, usually represent:
controller/action/args/
- The first segment represents the controller class that should be invoked.
- The second segment represents the controller function, or method, that should be called.
- The third, and any additional segments, represent the ID and any variables that will be passed to the controller function.
PHPRad Router Very Smart
Varous Examples of Routing. We are going to use a project called myshop
To Load Product List Page
GET - http://localhost/myshop/products
or
GET - http://localhost/myshop/products/index
To Load Custom Product List Page
GET - http://localhost/myshop/products/active_products
To Load Product List Page By Property value
GET - http://localhost/myshop/products/category/plastics
or
GET - http://localhost/myshop/products/index/category/plastics
To Load a Product Detail Page
GET - http://localhost/myshop/products/2
or
GET - http://localhost/myshop/products/view/1
To Display Add Product Form Page
GET - http://localhost/myshop/products/add
To Save Product
POST - http://localhost/myshop/products/add
To Display Edit Product Form Page
GET - http://localhost/myshop/products/edit/3
To Update Product Changes
POST - http://localhost/myshop/products/edit/3
Controllers
PHPRad Controllers are of two categories- The System Controller (system/BaseController.php)
- Application Controllers (app/controllers/*)
Base Controller
The Base Controller contains two classes
-
BaseController class
This is the main controller class which other controllers can extends from. -
SecureController class
This class also extends to the BaseController Class
Other application Controllers can also extends from this class if they are to be authenticated and authorise before executing any of the controller actions.
You don't need to add authorisation code on all you controller actions if you extends to SecureController
Application Controllers
The application Controllers are stored in the app/controllers directory.
The controller filename must be the same with the controller class name.They must end with Controller
Also, always make sure your controller extends the parent controller class so that it can inherit all its methods.
// app/controllers/ProductsController.php
class ProductsController extends BaseController{
function index( $arg1 = null , $arg2 = null ){
}
}
Controller Naming Conventions
A Controller is simply a class file that is named in a way that can be associated with a URI.
Consider this URI:
http://localhost/myshop/products
In the above example, PHPRad would attempt to find a controller named ProductsController.php and load it.
Important
Class names must start with an uppercase letter.
Also, always make sure your controller extends the parent controller class (BaseController , SecureController) so that it can inherit all its methods.
Controller Actions | Methods
In the above example the method name is index(). The “index” method is always loaded by default if the second segment of the URI is empty. Another way to show your “Hello World” message would be this:
The second segment of the URI determines which method in the controller gets called.
Passing URI Segments to your methods
If your URI contains more than two segments they will be passed to your
method as parameters.
// app/controllers/ProductsController.php
class ProductsController extends BaseController{
/*
http://localhost/myshop/products
or
http://localhost/myshop/products/index
*/
function index( $arg1 = null , $arg2 = null ){
}
//http://localhost/myshop/products/edit/8
function edit( $rec_id = null){
}
//http://localhost/myshop/products/add
function add( ){
}
/*
http://localhost/myshop/products/view/8
or
http://localhost/myshop/products/8
*/
function view( $rec_id = null ){
}
//http://localhost/myshop/products/delete/8
function delete( $rec_id = null){
}
//http://localhost/myshop/products/custom_action/category/plastics/status/active
function custom_action( $arg1 = null , $arg2 = null, $arg3 = null, $arg4 = null ){
}
}
Defining a Default Controller
PHPRad can be told to load a default controller when a URI is not present, as will be the case when only your site root URL is requested. To specify a default controller, open your config.php file and set this variable:
By Default "Index" is the Default ControllerExample
http://localhost/myshop/
This will load the default controller
Private methods
In some cases you may want certain methods hidden from public access. In order to achieve this, simply declare the method as being private or protected and it will not be served via a URL request. For example, if you were to have a method like this:
Trying to access it via the URL, like this, will not work:
Rendering Output
PHPRad has a BaseView class that takes care of sending your final rendered data to the web browser automatically. More information on this can be found in the Views Section of this page
You can render output in PHPRad using the view class render methodController Render Statement:
$this -> view -> render( 'viewpath/viewname.php' , $model_data , "layoutname.php");
Basic Example:
class ProductsController{
function add( $rec_id = null ){
$this -> view -> render( 'products/add.php');
}
}
Basic Example: Passing Model Data to the view
class ProductsController{
function view( $rec_id = null ){
$model = new ProductsModel;
$model_data = $model -> view( $rec_id );
$this -> view -> render( 'products/view.php' , $model_data );
}
}
Basic Example: Passing Additional Data to the view and the layout
class ProductsController{
function active_products( $rec_id = null ){
//Data can come from database
$view_data=array(
"total_records" => 30 ,
"records" => array("data1","data2","data3")
);
// You can pass more data to view and layout.
$this -> view -> page_title = "Actives Products";
$this -> view -> product_categories = array( "Plastics" , "Iron" , "Ceramics" , "Furniture" );
$this -> view -> render( 'products/list.php' , $view_data);
}
}
Basic Example: Specifying Custom Layout layout
class ProductsController{
function active_products( $rec_id = null ){
$model = new ProductsModel;
$view_data = $model -> view( $rec_id );
$this -> view -> render( 'products/view.php' , $view_data , "view_layout.php");
}
}
Views
A view is simply a web page, or a page fragment, like a header, footer, sidebar, etc. In fact, views can flexibly be embedded within other views (within other views, etc., etc.) if you need this type of hierarchy.
Views are not loaded directly via the url request, they are loaded by the controller when a request is made.
The controller is responsible for fetching a particular view. If you have not read the Controllers page you should do so before continuing.
How to get model data passed by the controller in a layout view or partial view
<?php
$view_data = $this -> view_data;
$page_title = $this -> page_title;
$product_categories = $this -> product_categories;
?>
In PHPRad There are two main categories of view
-
Layout Views
The layout view load main view and other views
-
Partial Views
The partial views are loaded onto the layout page
The Layout View
Sample of PHPRad Layout View
<?php
$page_title = $this -> page_title; //Page Data From Controller
?>
<!DOCTYPE html>
<html>
<head>
<?php
// using Html helper class to load head tags
Html :: page_title($page_title);
Html :: page_meta('author',META_AUTHOR);
Html :: page_meta('keyword',META_KEYWORDS);
Html :: page_meta('description',META_DESCRIPTION);
Html :: page_meta('viewport',META_VIEWPORT);
Html :: page_css('material-icons.css');
Html :: page_css('animate.css');
Html :: page_css('bootstrap-theme-flatly.css');
Html :: page_css('custom-style.css');
?>
<?php
Html :: page_js('jquery-2.js');
?>
</head>
<body>
<?php
//Render Page header and main navbar
Html :: page_header();
?>
<div id="main-content">
<?php
// Render page view(Main Partial view);
$this->render_body();
?>
</div>
<?php
//Render page footer
Html :: page_footer();
?>
<?php
Html :: page_js('bootstrap.js');
?>
</body>
</html>
The Partial View
Sample of PHPRad Partial View
<?php
$view_data = $this -> view_data; //Page Data From Controller
$records = $view_data -> records;
$total_records = $view_data -> total_records;
?>
<section class="page animated fadeIn">
<div class="page-header">
<h4>Products</h4>
</div>
<?php
if( !empty( $records ) ){
foreach( $records as $data ){
?>
... output here
<?php
}
}
else{
?>
<div class="text-danger">
<h4> No Record Found</h4>
</div>
<?php
}
?>
</section>
Rendering multiple views in a layout or another view
PHPRad has three main base method which you can use to render multiple views on another another view or layout:
-
render_body() method
Render main page view from the controller onto the layout
-
render_page($page_url , $optional_viewname) method
Render page as a partial view onto another page using url parameters
You can specify optional view which will handle the view data
-
load_view() method
Include view onto another view or layout as a partial view
<?php
$page_title = $this -> page_title; //Page Data From Controller
?>
<!DOCTYPE html>
<html>
<head>
<?php
// using Html helper class to load head tags
Html :: page_title($page_title);
Html :: page_meta('author',META_AUTHOR);
Html :: page_meta('keyword',META_KEYWORDS);
Html :: page_meta('description',META_DESCRIPTION);
Html :: page_meta('viewport',META_VIEWPORT);
Html :: page_css('material-icons.css');
Html :: page_css('animate.css');
Html :: page_css('bootstrap-theme-flatly.css');
Html :: page_css('custom-style.css');
?>
<?php
Html :: page_js('jquery-2.js');
?>
</head>
<body>
<?php
//Render Page header and main navbar
Html :: page_header();
?>
<div id="main-content">
<?php
// Render page view - main partial view;
$this->render_body();
?>
<section class="page animated fadeIn">
<div class="row">
<div class="col-sm-6">
<?php
// Page url with query string
$this -> render_page( 'products/sold_products?orderby=date_sold&ordertype=desc&limit_count=10' );
?>
</div>
<div class="col-sm-6">
<?php
// Using a different view to handle the page data
$this -> render_page( 'products' , 'products/gridview.php' );
?>
</div>
</div>
</section>
<section class="page animated fadeIn">
<div class="row">
<div class="col-sm-6">
<?php
$this -> load_view( 'components/barchart.php' );
?>
</div>
<div class="col-sm-6">
<?php
// Passing data to the view
$page_heading="This is page heading";
$this -> load_view( 'components/barchart.php' , $page_heading );
?>
</div>
</div>
</section>
</div>
<?php
//Render page footer
Html :: page_footer();
?>
<?php
Html :: page_js('bootstrap.js');
?>
</body>
</html>
Model
What is a Model?
Models are PHP classes that are designed to work with information in your database. For example, let’s say you use PHPRad to manage a blog. You might have a model class that contains functions to insert, update, and retrieve your blog data. Here is an example of what such a model class might look like:
Models are optionally available for those who want to use a more traditional MVC approach.
Anatomy of a Model
Model classes are stored in your app/models/ directory.
The basic prototype for a model class is this:
class ProductsModel extends BaseModel {
function load( $fieldname = null , $fieldvalue = null ) {
}
function view( $rec_id = null ) {
}
function add( $modeldata ) {
}
function edit( $rec_id , $modeldata=null ) {
}
function delete( $rec_ids ) {
}
}
Where ProductsModel is the name of your class. Class names must have the first letter capitalized with the rest of the name lowercase prefixed with Model. Make sure your class extends the BaseModel class.
The file name must match the class name. For example, if this is your class:
class ProductsModel extends BaseModel {
}
Your file will be this:
app/models/ProductsModel.php
Some Basic Crude Operations Using Mysqli ORM Library By default PHPRad comes with this library
class ProductsModel extends BaseModel {
function load( $fieldname = null , $fieldvalue = null ) {
$fields = array(
"product_id",
"title",
"description",
"category",
"price",
"photo"
);
$limit = $this -> get_page_limit(); // return limit from BaseModel Class e.g array(5,20)
//if search query request
if(!empty($this -> search)){
$text = $this -> search;
$this -> db -> orWhere ( 'product_id' , "$text" , '=' );
$this -> db -> orWhere ( 'title' , "%$text%" , 'LIKE' );
$this -> db -> orWhere ( 'description' , "%$text%" , 'LIKE' );
}
//if orderby is set in the request
if(!empty( $this -> orderby )){
$this -> db -> orderBy($this->orderby,$this->ordertype);
}
//if record filter by table field name and value is set
if(!empty($fieldname)){
$this -> db -> where( $fieldname , $fieldvalue );
}
$tc = $this ->db -> withTotalCount();
$arr= $this ->db -> get( 'products' , $limit, $fields );
return array( "result" => $arr , "totalcount" => $tc -> totalCount );
}
function view( $rec_id = null ) {
$fields = array(
"product_id",
"title",
"description",
"category",
"price",
"photo"
);
$this -> db -> where( 'product_id' , $rec_id );
$obj = $this -> db -> getOne( 'products', $fields );
return $obj;
}
function add( $modeldata ) {
$result=array();
$rec_id = $this -> db -> insert( 'products' , $modeldata );
if( $rec_id ){
$result[ 'rec_id' ] = $rec_id;
$result[ 'error' ] = null;
}
else{
$result[ 'rec_id' ] = null;
$result[ 'error' ] = $this -> db -> getLastError();
}
return $result;
}
/**
* edit Model Action
* if $modeldata is empty get and return edit data else save $modeldata
* @param $modeldata array
* @param $rec_id value
* @return array
*/
function edit( $rec_id , $modeldata=null ) {
if(!empty($modeldata)){
$result = array();
$this -> db -> where ( 'product_id' , $rec_id );
$bool = $this-> db-> update('products',$modeldata);
if( $bool ){
$result['rec_id'] = $rec_id;
$result['error'] = null;
}
else{
$result['rec_id'] = null;
$result['error'] = $this -> db -> getLastError();
}
return $result;
}
else{
$fields = array(
"product_id",
"title",
"description",
"category",
"price",
"photo"
);
$this -> db -> where('product_id' , $rec_id);
$obj = $this -> db -> getOne ('products' , $fields);
return $obj;
}
}
/**
* delete Model Action
* Support multiple record delete when records id are separated by comma (,)
* @param $rec_id
* @return boolean
*/
function delete( $rec_ids ) {
$arr_id = explode(",",$rec_ids);
foreach($arr_id as $rec_id){
$this->db->where('product_id' , $rec_id,"=",'OR');
}
$bool = $this->db->delete('products');
return $bool;
}
function custom_query( $arg1 = null , $arg1 = null ) {
$arr = $this -> db -> rawQuery("SELECT * FROM products WHERE status=? AND category=?", array($arg1, $ar2 ));
return $arr;
}
}
Libraries
When we use the term Libraries we are normally referring to the classes or packages that are located in the lib directoryIn summary:
- You can create entirely new libraries.
- You can extend system libraries.
- The page below explains these three concepts in detail.
Note
Your library classes should be placed within your lib directory, as this is where PHPRad will look for them when they are initialized.
Naming Conventions
- File names must be capitalized. For example: Myclass.php
- Class declarations must be capitalized. For example: class Myclass
- Class names and file names must match.
PHPRad Libraries
Anti XSS library
Cross Site Scripting Prevention ClassPHPRad comes with a Cross Site Scripting prevention filter, which looks for commonly used techniques to trigger JavaScript or other types of code that attempt to hijack cookies or do other malicious things. If anything disallowed is encountered it is rendered safe by converting the data to character entities.
To filter data through the XSS filter use the xss_clean($content) method:
$clean_data = xss_clean($content);
CSRF
Cross Site Scripting Prevention ClassPHPRad Csrf Class is auto instantiated.
Csrf Highly Ransomised Token is generated once and save in a session
Csrf token can be passed to a controller action as a GET or POST Request.
Example of passing token to a request
<a href="<?php print_link('products/delete/2?csrf_token='. Csrf :: $token); ?>">Csrf Protect Resurce</a>
<input type="hidden" name="csrf_token" value="<?php echo Csrf :: $token; ?>" />
To check for csrf use the Csrf :: cross_check function in the controller action before any other script.
Exampleclass ProductsController extends BaseController{
function add() {
Csrf :: cross_check();
// code continues here
}
}
Page Access Manager Library (libs/PageAccessManager.php)
Role Based Access Control(RBAC) is a model in which roles are created for various job functions, and permissions to perform certain operations are then tied to roles. A user can be assigned one or multiple roles which restricts their system access to the permissions for which they have been authorized
Defining Users roles Page Access
public static $usersRolePermissions = array(
"administrator" =>
array(
"products" => array('list' , 'view'),
"clients" => array('list', 'view' , 'add' , 'edit' , 'delete')
"users" => array('list' , 'view' , 'add' , 'edit' , 'delete')
),
"manager" =>
array(
"products" => array('list' , 'view' , 'add' , 'edit' , 'delete'),
"clients" => array('list' , 'view'),
"users" => array('list' , 'view' , 'add')
),
"cashiers" =>
array(
"products" => array('list','view'),
"clients" => array('list','view','add','edit','delete')
)
);
Example
public static $usersRolePermissions = array(
"administrator" => "*"
);
To disabled Page Access manager
public static $usersRolePermissions = "*";
Upload
PHPRad file upload handler
Support Multi File Upload
Return File Paths separated by comma
Simple upload handler
$filepaths="";
if(!empty($_FILES['photo'])){
$uploader=new Uploader;
$upload_config = array(
'maxSize' => 3,
'limit' => 1,
'extensions' => array('jpg' , 'png' , 'gif' , 'jpeg'),
'uploadDir' => 'uploads/files/',
'required' => false,
'returnfullpath' => true
);
$upload_data = $uploader -> upload( $_FILES['photo'] , $upload_config );
if($upload_data['isComplete']){
$filepaths = $upload_data['data']['files'];
}
if($upload_data['hasErrors']){
$errors = $upload_data['errors'];
foreach($errors as $key => $val){
//you can pass upload errors to the view
$this -> view -> form_error[$key] = $val;
}
}
}
}
Form | Data Validation
PHPRad file upload handler
PHPRad Support Both Server Side Validation and client Side Validation
The client validation relies on HTML 5 control validation. No Additional javascript plugin is included
The server side validation uses the validation library libs/Validation.php
Sample of validation data
$postdata = transform_request_data($_POST);
$rules_array = array(
'customer_name'=>array('type'=>'string', 'required'=>true, 'min'=>0, 'max'=>50, 'trim'=>true,'error_msg'=>''),
'telephone'=>array('type'=>'tel', 'required'=>false, 'min'=>0, 'max'=>50, 'trim'=>true,'error_msg'=>''),
'range'=>array('type'=>'numeric', 'required'=>false, 'min'=>0, 'max'=>50.6, 'trim'=>true,'error_msg'=>''),
'order_no'=>array('type'=>'number', 'required'=>false, 'min'=>0, 'max'=>50, 'trim'=>true,'error_msg'=>''),
'date'=>array('type'=>'date', 'required'=>false, 'min'=>0, 'max'=>64000, 'trim'=>true,'error_msg'=>''),
'website_url'=>array('type'=>'url', 'required'=>false, 'min'=>0, 'max'=>50, 'trim'=>true,'error_msg'=>''),
);
$val=new Validation;
$val->addSource($postdata);
$val->addRules($rules_array);
$val->run();
if(!empty($val->errors)){
$this->view->form_error=$val->errors;
$this->view->render('delivery/add.php' ,null,'form_layout.php');
return null;
}
$modeldata=$val->sanitized;
Helpers
Helper FunctionsHelpers, as the name suggests, help you with tasks. Each helper file is simply a collection of functions in a particular category. There are Html Helpers, that assist in rendering page components, there are Image Helpers that help you in manipulating images(resize,crop), Menu Helpers helps in building different styles of both dynamic and static menu items, Mailer Helpers help you deal with email, etc.
Helpers create an easy inteface in which core system class and other other external libraries functionalities can easily be accessed
Unlike most other systems PHPRad Helpers are not written in an Object Oriented format. They are simple, procedural functions. Each helper function performs one specific task, with no dependence on other functions.
PHPRad helpers are autoloaded.
Helpers are typically stored in your helpers directory.
PHPRad Default helpers
Html helper
Html help helps is building reusable view components.
-
page_title ( $title )
Display page head title <title>Title goes here</title><?php Html :: page_title("The title of the page goes here"); ?>
-
page_meta ( $name , $content=null)
Display Html Head Meta Tag <meta name="" content="" /><?php Html :: page_title("The title of the page goes here"); ?>
-
page_css ( $filename)
include css file. the file must be under assets/css directory<link href="" type="stylesheet" /><?php Html :: page_title("bootstrap.min.css"); ?>
-
page_js ( $filename=null)
Include javascript file. The file must be under assets/js directory <script src="" ></script><?php Html :: page_js("bootstrap.min.js"); ?>
-
page_img ( $imgsrc , $resizewidth=null , $resizeheight=null , $link=null , $class=null , $attrs=null)
Display Html Image Tag.
Can be Use to Display Multiple Images Separated By Comma
Also Can Be Use To Resize Image Via Url
Images are cached <img class="" src="" /><?php Html :: page_img("assets/images/pic1.jpg",50,50); ?>
-
page_header ( $arg = null )
Display renders the application header including the menu bar<?php Html :: page_header(); ?>
-
render_menu ( $arrMenu , $menu_class='nav navbar-nav', $submenu_class='dropdown-menu')
Display builds and render navigation menu for the menu items
Build Menu List From Array
Support Multi Level Dropdown Menu
include Font icon Supported
Set Active Menu Base on The Current Page || url<?php $arr_menu = array( array( 'path' => 'products', 'label' => 'Products List', 'icon' => 'item' ), array( 'path' => 'users', 'label' => 'Manage Users', 'icon' => 'user' ), ); Html :: render_menu($arr_menu); ?>
//Sample output <ul class="nav navbar-nav"> <li> <a href="products"> <i class="material-icons"></i> Products </a> </li> <li class="active"> <a href="users"> <i class="material-icons"></i> Manage Users </a> </li> </ul>
-
page_breadcrumb ()
Display the page bread crumbs links<?php Html :: page_breadcrumb(); ?>
-
export_component ()
Display Export Controls for the current page<?php Html :: export_component(); ?>
-
page_footer ( $arg = null )
Display Page Footer view<?php Html :: page_footer(); ?>
Here are some of the html helper
Pagination helper
Pagination helper is used to build and render pagination for a page.
PHPRad Pagination helpers comes with different customization to help you build different style and layout of pagination
Pagination helper are used inside a view
Example of pagination helper
<?php
$pager = new Pagination( $total_records , $record_count );
$pager ->show_page_count = true;
$pager ->show_record_count = true;
$pager ->show_page_limit = true;
$pager ->show_page_number_list = true;
$pager ->pager_link_range = 5;
$pager->render();
?>
You can decide to hide any of the pagination component. for individual page

Mail helper
This is used to send mail using SMTP or normal mail function.
To use mail you must edit the default mail configuration settings in config.php file
Uses PHPMailer Library
Sample of Mail Sending<?php
$user_name = "username";
$receiver_email = "useremail@gmail.com";
$mail_title = "Email Address Verification";
$mail_body = "Mail body goes here. html content is also allowed";
$mailer = new Mailer;
$mailer ->sender_email="senderemail@site.com";
$mailer ->sender_name="sender name";
$mailer ->send_mail($receiver_email , $mail_title , $mail_body);
?>
Global Functions helper
PHPRad function helper are some some common function which are used to accomplish some task..
Here are some functions to use on any page or class.
-
get_active_user($field=null)Get Logged In User Details From The Session
Get particular field value of the active user otherwise return array of active user fieldsReturn Value Type: array | stringecho get_active_user('user_city'); -
user_login_status()Check if there is active User Logged In
Return Value Type: boolean
-
authenticate_user()Authenticate And Check User Page Access Eligibility
Return Value Type: Redirect to Login Page Or Displays Error Message When user access control authorization Fails -
is_mobile()Check if current browser platform is a mobile browser
Can Be Used to Switch Layouts and Views on A Mobile PlatformReturn Value Type: boolean -
print_link($path=null)Echo Absolute Url Address of a pathExample : <a href="<?php print_link('users/add'); ?>">Add Users</a>
-
set_url($path=null)Return Absolute Url Address of a path
-
set_img_src($imgsrc,$width=null,$height=null)Convinient Function To Resize Image Via Url of the Image Src if the src is from the same origin then image can be resizeExample : <img src="<?php echo set_img_src('uploads/images/89njdh4533.jpg',50,50); ?>" />;
-
datetime_now()will return current DateTime in Mysql Default Date Time Format (Y-m-d H:i:s)Example : 2017-02-13 03:15:00
-
time_now()will return current Time in Mysql Default Date Time Format (H:i:s)Example : 03:15:00
-
date_now()will return current Date in Mysql Default Date Time Format (Y-m-d)Example: 2017-02-13
-
relative_time($date=null)Parse Date Or Timestamp Object into Relative TimeExample : 2 days ago 2 days from now
-
human_date($date=null)Parse Date Or Timestamp Object into Human Readable DateExample : 26th of March 2016
-
random_chars($limit=12,$context='...')Generate a Random String and characters From Set Of supplied data contextExample : f#8Gt4!a@hdt
-
random_str($limit=12,$context='...')Generate a Random String From Set Of supplied data contextExample : Ga89KJ67adf4
-
random_num($limit=6,$context='1234567890')Generate a Random Number From Set Of Supplied NumbersExample : 681423
-
random_color($alpha=1)Generate a Random color StringExample : rgba(230,12,9,0.5)
-
set_flash_msg($msg,$type="success",$dismissable=true,$showduration=5000)Set Msg that Will be Display to User in a Session.
Can Be Displayed on Any View. -
show_flash_msg()Display The Message Set In MsgFlash Session On Any Page.
Will Clear The Message Afterwards -
redirect_to_page($path=null)Convinient Function To Redirect to Another PageExample : redirect_to_page("users/view/".USER_ID);
-
redirect_to_action($action_name=null)Convinient Function To Redirect to Page ActionExample redirect_to_action('add');